#include <iostream>
#include <opencv2/core.hpp>
#include <opencv2/imgcodecs.hpp>
using namespace std;
using namespace cv;
class MatRef
{
public:
inline MatRef(int rows, int cols, uchar (*data)[3]);
static void ShowMatrix(const string&& str, const Mat& mat);
inline void ShowMatrix(const string&& str) const;
inline void RefCount() const;
inline Mat& operator=(const Mat& rhs);
inline Mat GetMatrix() const;
inline Mat& GetMatrixRef();
inline Mat LocationRoi(Range&& rowRange, Range&& colRange);
inline Mat LocationRoi(Rect&& rect);
private:
Mat mat;
};
MatRef::MatRef(int rows, int cols, uchar (*data)[3]);
{
// mat = (Mat_<double>(2, 3) << 1, 2, 3, 4, 5, 6);
for (int i = 0; i < rows; ++i)
mat.push_back(move(Mat(1, cols, CV_8UC1, data + i)));
}
void MatRef::ShowMatrix(const string&& str) const
{
cout << str << endl << mat << endl;
}
void MatRef::ShowMatrix(const string&& str, const Mat& mat)
{
cout << str << endl << mat << endl;
}
void MatRef::RefCount() const
{
cout << "The ref count of matrix = " << mat.u->refcount << endl;
}
Mat& MatRef::operator=(const Mat& rhs)
{
return mat = rhs;
}
Mat MatRef::LocationRoi(Rect&& rect)
{
ShowMatrix("matrix = ");
Mat roi(mat, rect);
ShowMatrix("roi = ", roi);
cout << "rect = " << rect << endl;
return roi;
}
Mat MatRef::LocationRoi(Range&& rowRange, Range&& colRange)
{
Size wholeSize;
Point pt;
Mat roi = mat(rowRange, colRange);
ShowMatrix("matrix = ");
roi.locateROI(wholeSize, pt); // 定位感兴趣的区域
cout << "whole size = " << wholeSize << endl;
cout << "offset point = " << pt << endl;
ShowMatrix("roi = ", roi);
return roi;
}
Mat MatRef::GetMatrix() const
{
return mat.clone();
}
Mat& MatRef::GetMatrixRef()
{
return mat;
}
int main()
{
uchar arr[][3] = { { 1, 2, 3 }, { 4, 5, 6 } };
uchar(*ptr)[3] = arr; // 行指针指向多维数组时不需要取多维数组的地址, 一维数组必须取地址
MatRef a(2, 3, ptr);
a.ShowMatrix("matrix = ");
a.RefCount();
Mat b = a.GetMatrixRef();
a.RefCount();
Mat c = a.GetMatrix();
a.RefCount();
// 利用行列区间定位感兴趣的区域
int rowStart = 0, rowEnd = 1, colStart = 1, colsEnd = 3;
a.LocationRoi(Range(rowStart, rowEnd), Range(colStart, colsEnd));
// 根据矩阵中的一个点的坐标和size范围定位感兴趣的区域
Point pt(1, 0);
int with = 2, height = 1;
a.LocationRoi(Rect(pt.x, pt.y, with, height));
Mat d = a.GetMatrixRef();
a.RefCount();
cout << "if matrix d is refference of matrixRef then d.row(1) = d.row(0) + d.row(1)" << endl;
d.row(1) = d.row(0) + d.row(1);
MatRef::ShowMatrix("matrix d = ", d);
a.RefCount();
Mat e = a.GetMatrixRef();
a.RefCount();
Mat t(e.col(1) + e.col(2));
t.copyTo(e.col(0));
MatRef::ShowMatrix("matrix e = ", e);
a.RefCount();
return 0;
}
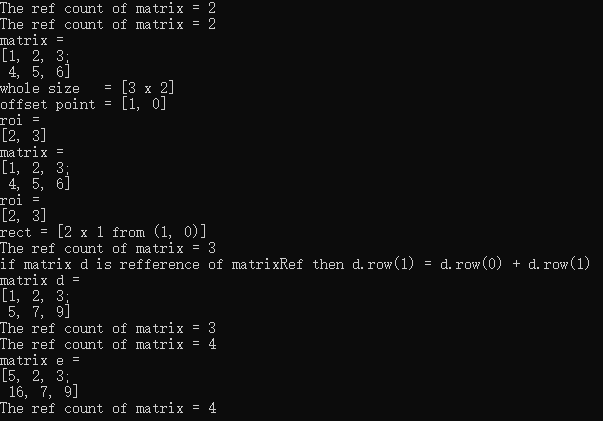